FiboFilters triggers a series of JavaScript events that allow you to interact with and customize the filter elements or the rendered product grid. Below is a list of all the available events and their descriptions, along with examples on how to use them.
Examples
Example 1
Display a message in the browser console when the products are reloaded after filtering.
<script> if (typeof fiboFilters !== 'undefined') { function fibofiltersGridReloaded() { console.log('Grid was reloaded'); } fiboFilters.hooks.addAction( 'fiboFilters.renderer.products_loaded', 'fibofilters', fibofiltersGridReloaded ); fiboFilters.hooks.addAction( 'fiboFilters.renderer.product_placeholders_overwritten', 'fibofilters', fibofiltersGridReloaded ); } </script>
Example 2
Display a message in the browser console when product grid rendering is complete.
<script> if (typeof fiboFilters !== 'undefined') { fiboFilters.hooks.addAction('fiboFilters.renderer.completed', 'fibofilters', () => { console.log('FiboFilters UI was rendered.'); }); } </script>
Example 3
Display a message in the browser console when filters are displayed and show the current layout.
<script> if (typeof fiboFilters !== 'undefined') { fiboFilters.hooks.addAction('fiboFilters.renderer.filters_displayed', 'fibofilters', (layout) => { console.log(`Hook fired: fiboFilters.renderer.filters_displayed, layout: ${layout}`); }); } </script>
Example 4
Display a message in the browser console when filters are applied and the state is changed. The code also displays the change that’s taken place, together with the previous and new states.
<script> if (typeof fiboFilters !== 'undefined') { fiboFilters.hooks.addAction('fiboFilters.core.state_updated', 'fibofilters', (changes, oldState, newState) => { console.log(`Hook fired: fiboFilters.core.state_updated. changes,oldState,newState:`, changes, oldState, newState); }); } </script>
Example 5
Add custom HTML into the product grid. The example below will add HTML after every 4th product.
Note:
Inserting HTML into the product grid can break the layout in some themes. To avoid this, use the fiboFilters.renderer.fix_product_classes
hook after modifying the grid.
<script> if (typeof jQuery !== 'undefined' && typeof fiboFilters !== 'undefined') { fiboFilters.hooks.addAction('fiboFilters.renderer.products_loaded', 'fibofilters', () => { jQuery('.products.columns-3 li.my-custom-product').remove(); // Insert custom HTML after every 4th product in loop with selector `.products.columns-3` jQuery('.products.columns-3 li.product:nth-child(4n)').after('<li class="product my-custom-product"><div><img src="<?php echo wc_placeholder_img_src(); ?> " /><h3>My custom HTML</h3></div></li>'); fiboFilters.hooks.doAction('fiboFilters.renderer.fix_product_classes'); }); } </script>
The effect is depicted in the screenshot below:
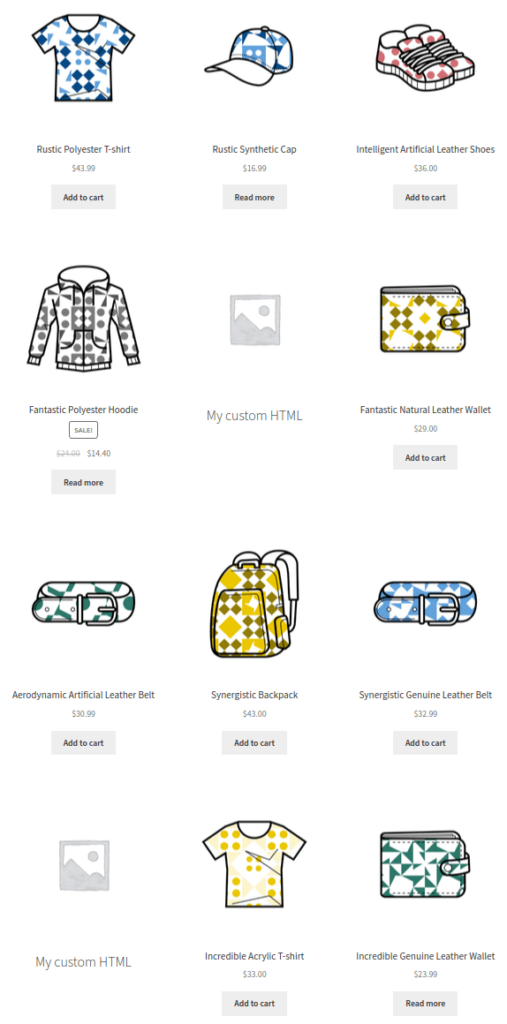