Allow users to filter content based on a numerical field value. This facet might consist of three elements: “max” and “min” input boxes, a slider, and a radio range presented as a “radio” button. In the settings, you can decide what elements will be displayed.
Table of Contents
- Available options
- Number format
- Customizing min and max labels in sliders
- Changing currency settings
- Normalizing units for consistent filtering
The Range filter type is available only for filters where the data source consists of numeric values. Mixed numbers and letters or special characters, like “100 cm”, “8kg”, “60%”, “$89,99”, are still treated as numeric.
FiboFilters analyzes the data set and determines the prefix, suffix and decimal precision for the filters. In case of mixed numbers and letters, only the number is indexed and the string part is stored as a prefix or suffix for the filter (depending on its position).
If a filter has values with different units, like “50cm”, “75cm”, “1m”, FiboFilters won’t treat this filter as numeric and the Range type will be unavailable. To work around this, read the “Normalizing units for consistent filtering” section below.
This filter type will be selected automatically if you pick “Price” as the filter source.
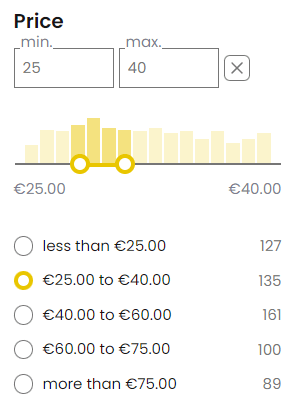
Available options
Number format
General settings for numeric filters
The price format is automatically determined by the settings in WooCommerce
> Settings
> General
> Currency options
.
For values other than price, the thousands separator and decimal point are pulled from the global variable $wp_locale
. These can be overridden using the following PHP snippet:
add_filter( 'fibofilters/config/number_format', function ( $number_format ) { $number_format['thousands_sep'] = ' '; $number_format['decimal_point'] = ','; return $number_format; } );
ⓘLearn how to add this snippet to your WordPress.
You can also modify the number format to include a prefix, suffix, and precision:
add_filter( 'fibofilters/config/number_format', function ( $number_format ) { $number_format['thousands_sep'] = ' '; $number_format['decimal_point'] = ','; $number_format['precision'] = 2; $number_format['prefix'] = '>'; $number_format['suffix'] = '<'; return $number_format; } );
ⓘLearn how to add this snippet to your WordPress.
Precision can be set to -1
. This will make FiboFilters display the number of digits after the decimal point that was defined for the number while indexing.
Setting number format for specific filters
By default, FiboFilters will format the numbers according to global settings: WooCommerce settings, the $wp_locale
global variable or settings defined with the fibofilters/config/number_format
filter.
To override these settings for a specific filter, go to the filter’s “Advanced settings”, disable the “Use global number formatting” option and you’ll be able to populate the prefix, suffix and precision for the given filter:
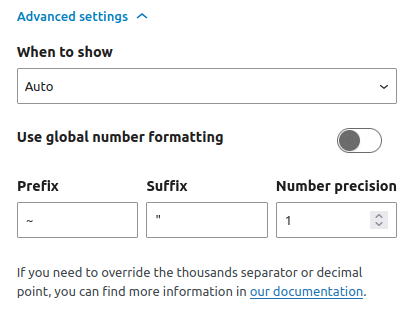
This, in turn, can be overridden with a code snippet. The example below will modify the format for the “caps-size” filter, overriding the values from the filter’s settings:
add_filter( 'fibofilters/config/overrides', function () { return [ 'caps-size' => [ 'number_format' => [ 'prefix' => '~', 'suffix' => '"', 'precision' => 1, ] ], ]; } );
ⓘLearn how to add this snippet to your WordPress.
The result looks like that:
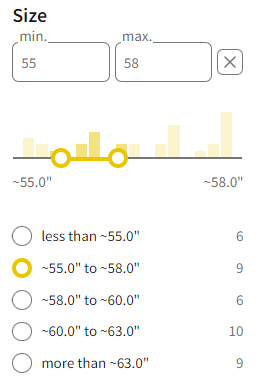
~
, suffix "
and number precision set to 1
. Customizing min and max labels in sliders
By default, the labels for min and max values in sliders are “min” and “max”. You can change these labels with the following snippets:
add_filter( 'fibofilters/config/range_slider_min_value_label', function() { return 'dynamic'; } ); add_filter( 'fibofilters/config/range_slider_max_value_label', function() { return 'dynamic'; } );
ⓘLearn how to add this snippet to your WordPress.
The allowed values are min
, max
, dynamic
and a number. dynamic
will automatically display the minimum/maximum value from the data set:
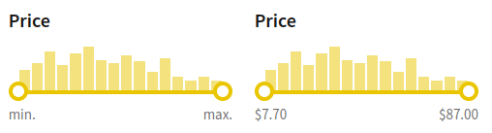
Customizing labels for specific filters
To change the min/max labels for a specific filter, such as the “caps-size” filter, use this code:
add_filter( 'fibofilters/config/overrides', function () { return [ 'caps-size' => [ 'range_slider_min_value_label' => 'dynamic', 'range_slider_max_value_label' => 'dynamic', ], ]; } );
ⓘLearn how to add this snippet to your WordPress.
Changing currency settings
FiboFilters use the same currency settings as WooCommerce. To change the settings, go to WooCommerce
> Settings
> General (tab)
> Currency options
section.
To change these settings only for the filters, a PHP filter is available. The snippet below changes the number of decimal places from two to zero. Additional settings, like symbol
or symbolPosition
, are also adjustable with this snippet.
add_filter( 'fibofilters/config/currency', function ( $currency ) { // All available options: // $currency = [ // 'code' => 'USD', // 'precision' => 2, // 'symbol' => '$', // 'symbolPosition' => 'left', // 'decimalSeparator' => ',', // 'thousandSeparator' => ' ', // 'priceFormat' => '%1$s%2$s' // ]; $currency['precision'] = 0; return $currency; } );
ⓘLearn how to add this snippet to your WordPress.
Normalizing units for consistent filtering
When working with numerical attributes that have mixed units (e.g., MB and GB), FiboFilters does not allow the use of the Range filter type. To enable range filtering, you need to normalize the units by converting all values to a common scale.
Example: normalizing storage capacity units
Suppose you have an attribute called pa_capacity
with the following terms:
- 128 MB
- 512 MB
- 1 GB
Since these values use different units, you can standardize them by converting 1 GB to 1024 MB. Use the following code snippet to achieve this:
add_filter( 'fibofilters/indexer/number_extended_overrides/source=tax_pa_capacity', function ( $map ) { $map['1 GB'] = '1024 MB'; return $map; } );
ⓘLearn how to add this snippet to your WordPress.
Note:
FiboFilters automatically prefixes taxonomy names with tax_
, meaning that pa_capacity
becomes tax_pa_capacity
in the hook. You can verify the correct source name in the filter details.
Once this code is applied, you will be able to use the Range filter type for the pa_capacity
attribute.
Handling non-numeric values
If your dataset includes non-numeric values such as “None”, you can map them to a numerical equivalent (e.g., 0 MB) to maintain compatibility with range filtering:
add_filter( 'fibofilters/indexer/number_extended_overrides/source=tax_pa_capacity', function ( $map ) { $map['None'] = '0 MB'; return $map; } );
ⓘLearn how to add this snippet to your WordPress.
By implementing these transformations, you ensure that all values remain comparable and filterable within FiboFilters.