After applying filters, WooCommerce typically requires a page reload to render new products. FiboFilters takes the process a few steps further: the products are rendered directly inside the catalog, right when the filter state is changed.
The main product HTML elements, typically stored within the ul.products > li.product
selector, are dynamically overwritten when the page reloads or when the filter state changes.
The HTML product template can be retrieved:
- From the source, rendered by WooCommerce during the standard page load
- Asynchronously, from the server for the products that haven’t been rendered with the first filtering query. For example, the products requested with the “Show more products” button or those that are presented after filter state changes
Key Benefits of AJAX product rendering
- No need for page reloads after changing filter states
- Instant product rendering – it offers an impeccable user experience
- HTML product templates are cached within the browser memory: the more customers filter, the fewer new templates need to be fetched.
WordPress themes support
Not every WP theme will work with our rendering engine out of the box. The main product wrapper could have a non-standard CSS selector and FiboFilters can’t recognize it. The same applies to some HTML product elements containing JavaScript elements attached.
Nonetheless, we decided that the pros largely outweigh the cons. We prepared dedicated integrations for the most popular and best themes. See the list of currently supported themes.
What if FiboFilters doesn’t support my theme?
If the theme you use in your store is popular, it’s highly likely that we can provide the integration quickly. Please, reach out to us at support@fibofilters.com
You can also try to solve the issue by yourself – it’s easier than it sounds. The most common problems arise from incorrect selectors or unhooked JavaScript actions. Review the list below and find solutions for these errors.
Fixing loop start selector
FiboFilters tries to determine the loop start selector by analyzing the content of the HTML returned by the function wc_get_template( 'loop/loop-start.php' )
.
If the attempt is unsuccessful, the plugin then tries to find the default ul.products
selector.
If both attempts fail, then you can manually override the loop start with a PHP snippet:
add_filter( 'fibofilters/config/products_loop_selectors', function ( $classes ) { $classes[] = '.your-products-selector'; return $classes; } );
ⓘLearn how to add this snippet to your WordPress.
This way you’ll make sure that the error is fixed.
Fixing product JS events
Some themes register JavaScript events on product HTML elements. To append products, FiboFilters clears the loop start container contents, and then inserts the new product HTML there. Some of the pre-registered JavaScript events may get lost in the process. If this is the case, you’ll need to manually register these JS events every time after the products are rendered.
Please review the below example from the Flatsome theme. After product rendering, features like “Quick view” and “Add to cart” stopped working. To fix this, it was necessary to register the events again after rendering all the products.
(function($) { if (typeof fiboFilters !== 'undefined' && typeof jQuery !== 'undefined') { function fibofiltersFlatsomeFix() { if (typeof Flatsome !== 'object') { return; } const $container = jQuery('.shop-container'); jQuery('.shop-container .quick-view-added').removeClass('quick-view-added'); Flatsome.attach('quick-view', $container); Flatsome.attach('add-qty', $container) } fiboFilters.hooks.addAction('fiboFilters.renderer.products_loaded', 'fibofilters', fibofiltersFlatsomeFix); fiboFilters.hooks.addAction('fiboFilters.renderer.product_placeholders_overwritten', 'fibofilters', fibofiltersFlatsomeFix); } }(jQuery));
Fixing product image placeholder
Before an AJAX request returns the HTML template of a product, a “Placeholder image” is displayed. It’s the same image that WooCommerce uses to display a placeholder product image. You can change it in WooCommerce -> Settings -> Products -> Placeholder image
.
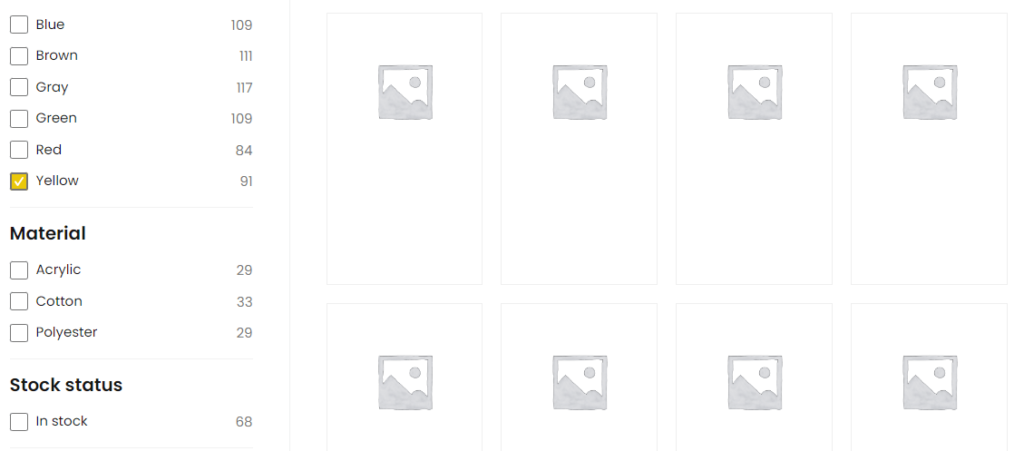
FiboFilters uses the following HTML structure to display the product placeholder:
<li class="product fibofilters-product-placeholder"> <div> <img src="<?php echo wc_placeholder_img_src(); ?>" /> </div> </li>
If this structure breaks the product grid, it can be adjusted to one more corresponding to the product HTML structure. This is an example of a PHP snippet that changes this HTML structure to a <div>
:
add_filter( 'fibofilters/request/html/product_placeholder', function () { ob_start(); ?> <div class="product fibofilters-product-placeholder col"> <div> <img src="<?php echo esc_url( wc_placeholder_img_src() ); ?> " alt="Placeholder image"/> </div> </div> <?php return (string) ob_get_clean(); } );
ⓘLearn how to add this snippet to your WordPress.
Endpoint URL for fetching missing products
Missing products are fetched using AJAX. The endpoint URL looks like this:
https://example.com/shop/?fibofilters-products=&ids=
In the ids
parameter, the product IDs whose templates need to be fetched are sent. If you’re on the shop archive page, /shop/
will be replaced with the archive page URL to preserve the context in which products are being loaded. Similarly, a different base URL will be used for this endpoint when products are being fetched on the search results page.
The URL of the endpoint can be changed using the following snippet:
add_filter( 'fibofilters/config/single_products_fetch_url', function ( $url ) { return home_url( '/shop/' ); } );
ⓘLearn how to add this snippet to your WordPress.
This endpoint returns the product data in HTML format wrapped within a JSON response. However, some page builders or themes (e.g., Elementor Loop Grid or Breakdance Builder) render product templates dynamically, which prevents FiboFilters from directly retrieving the product data.
In such cases, the endpoint will return the entire HTML of the page, allowing FiboFilters to extract the product information from it. Although this method is not ideal for performance, it is necessary for the proper functioning of FiboFilters when dynamic rendering is involved.
By default, this fix is enabled for certain themes and page builders. If you experience issues with rendering the product grid, you can manually enable this strategy by adding the following filter:
add_filter( 'fibofilters/config/products_fetching_strategy', fn() => 'page' );
ⓘLearn how to add this snippet to your WordPress.
This will ensure FiboFilters retrieves the necessary product data for proper display.